티스토리 뷰
In this article, we'll explore how to implement UART communications on Arduino boards using source code. We'll go through the process step-by-step, including how to connect the devices, configure the hardware serial module, and write the code to send and receive data over the UART connection.
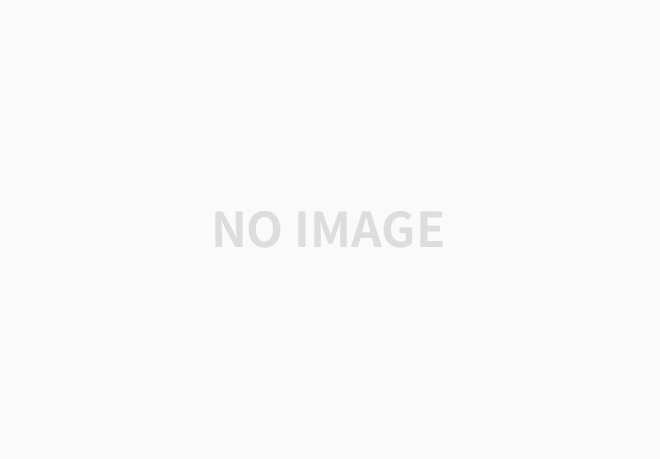
Connecting the Devices
The first step in implementing UART communication on Arduino boards is to connect the two devices to be used for communication. The exact number of pins and their usage varies depending on the specific Arduino board model, but the most common configuration is to use two pins: one for transmitting data (TX) and one for receiving data (RX).
To connect the devices, simply connect the TX pin on one device to the RX pin on the other, and vice versa.
Configuring the Hardware Serial Module
Once the devices are connected, the next step is to configure the hardware serial module on the Arduino board. This typically involves setting the baud rate and other communication parameters, such as the number of data bits and the parity setting.
The code to configure the hardware serial module is as follows:
void setup() {
Serial.begin(9600); // set the baud rate to 9600
}
Sending Data
With the hardware serial module configured, the next step is to write the code to send data over the UART connection. This is done using the Serial.write() function.
The code to send data is as follows:
void loop() {
char data = 'A'; // the data to be sent
Serial.write(data); // send the data
delay(1000); // wait 1 second before sending more data
}
Receiving Data
The final step is to write the code to receive data over the UART connection. This is done using the Serial.read() function.
The code to receive data is as follows:
void loop() {
if (Serial.available() > 0) { // check if there is data available to be read
char data = Serial.read(); // read the data
Serial.println(data); // output the data to the serial monitor
}
}
In this article, we've gone through the process of implementing UART communications on Arduino boards using source code. By following these steps, you'll be able to easily send and receive data between two devices using UART communication.
Whether you're looking to transfer data, debug your projects, or control other devices, UART is a versatile and powerful tool that is well-suited for use in a wide range of applications. So if you're looking to build projects using Arduino, be sure to make the most of the UART communication capabilities.